CS 2102 Homework Assignment #5
[not ready yet]
Assigned: Monday
November 27, 2006, 10:00
AM
Due: Tuesday December 5, 2006,
10:00 AM
*** new date: extra day on assignment ***
Guidelines:
Now
available.
The context for this homework assignment is a small game,
known by various names, including:
Amidakuji (阿
弥 陀 籤)
and Ghost Leg
(畫 鬼 腳).
For the purpose of this assignment, we will call the game
Amida. The goal of this assignment is to
produce a well-designed class that conforms to the behavioral interface provided
by the professor. You will validate the correct functioning of your classes by
means of JUnit test cases. In addition, I have provided a small GUI that can be
used to play the Amida game once your working classes are complete.
Description
Read the Amida
project description. To guide you in completing your solution, I have provided
you with a set of existing Java classes and interfaces. The goal is to design a class (and supporting classes) that
implements the provided amida.AmidaInterface. See the code example as found
in Examples/amida in the SourceForge/Eclipse public area. In the
following points, I break down the assignment of points for this assignment.
Initial Design
I believe you should adopt the following strategy.
- There can be any number of vertical lines on an Amida
board. Thus you will need to maintain a linked list of LineNodes, each of
which represents a vertical line.
- There can be any number of horizontal edges on an Amida
board. Because each edge connects two vertical lines, it may not be clear how
you should represent the information. I suggest that you consider each vertical
line to have a number of horizontal edges emanating from it to the right. Thus,
for the following Board:
you should store the following information:
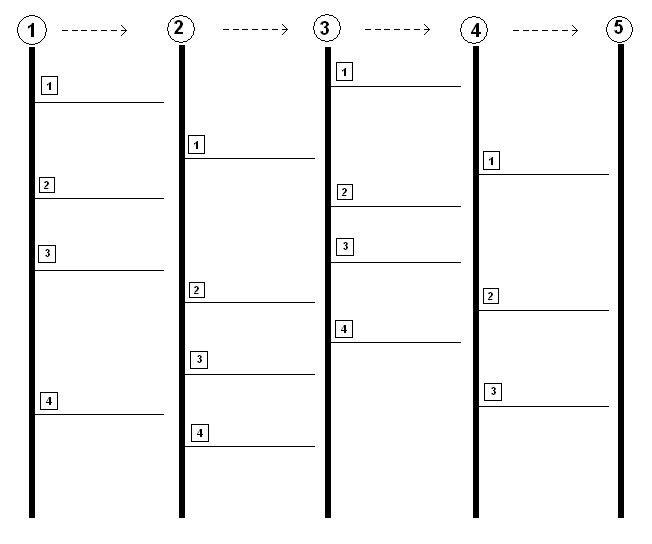
that is, where there is a linked list containing five LineNode objects. Each of
these LineNode objects is maintaining a linked list of EdgeNode objects. The
first three lines (reading from left to right) each has a list of four EdgeNode
objects, while the fourth vertical line has a list of three EdgeNode objects.
This project, then, is consumed by the idea of creating an
AmidaBoard class to maintain these lists of information in the face of requests
to:
- void addLine()
- boolean removeLine()
- void addEdge (int line, int verticalPos)
- boolean removeEdge (Edge edge)
- void removeAll()
Note that the vertical position starts at ZERO at the top
of the vertical line, and increases as you move vertically down the line. This
may seem counter-intuitive, but it is the standard for most computer graphics.
Once you are able to properly maintain this information,
you can implement the following requests:
- int numLines()
- int numEdges (int line)
- Edge getEdge (int line, int num)
Only then should you even contemplate attacking the two
final methods:
- boolean tooClose(int line, int verticalPos)
- int exitLine(int line)
Each of these methods is clearly defined within the
amida.AmidaInterface interface description file that is now available to you,
thus your AmidaBoard class must implement this interface. Your class must
provide a meaningful default constructor that takes no arguments.
Where do you start?
Watch the
HW5
skeleton video. Think about the
requirements that you are expected to implement. I would suggest that you
consider adding the following methods to LineNode:
- public boolean add (int verticalPos) throws
AmidaException -- Add an edge to the neighboring line to the right
- public void removeAll() -- Remove all horizontal
edges emanating from this line
- public boolean remove(int verticalPos) -- Remove the
horizontal edge emanating from this line to the right at the given vertical
position [if one exists]
- public boolean tooClose(int verticalPos) -- Would a
new edge emanating from the right of this line be too close to an existing
edge that already emanates to the right?
- public int numEdges() -- Return the number of edges
in the linked list of edges for this line
- public Edge getEdge(int n) -- Return the nth
edge from the top (n >= 1) emanating from this line
- public EdgeNode getEdgeAfter(int verticalPos) --
Return the EdgeNode (or null if none exists) which appears next vertically
Regarding the AmidaBoard class, the interface defines all
methods that you will need to provide. The exitLine(int line) method on
AmidaBoard is going to be a challenge to write: Consider the following
algorithm:
ExitLine algorithm:
Input: exitLine (int n)
Note that you model your edges to emanate to
the right from a vertical line
Output: an integer representing the line
which the Amida board exits when starting from line n
Algorithm:
// Start at the top of the line. Note that no
horizontal edge can have a verticalPos of 0.
verticalPos = 0
while (true) {
LineNode linenode = the LineNode representing the nth
line
LineNode prevnode = the LineNode representing the
(n-1)th line, if it exists
Edge leftEdge =
horizontal edge emanating from prevnode after verticalPos, if it
exists
Edge rightEdge = horizontal edge emanating from
linenode after verticalPos, if it exists
// Four cases to
consider.
// (a) if leftEdge and rightEdge are both null,
then you have found the exit: return n
// (b) if leftEdge and rightEdge are both not null,
then find closer one to verticalPos
// and
traverse it, updating n and verticalPos as needed.
// (c) if leftEdge is null, then traverse the
rightEdge, updating n and verticalPos
// (d) if rightEdge is null, then traverse the
leftEdge, updating n and verticalPos
// If we get here, we
must have had one of the cases (b), (c), or (d). We will loop back
// to execute the while loop again, with an updated n
value, and an updated verticalPos
// value, that makes forward progress on the board.
}
Analysis:
This algorithm is guaranteed to produce an
answer as long as the leftEdge and rightEdge actually have higher
verticalPos values than the verticalPos variable.
|
Point Allocation
-
[24 pts.] EdgeNode and LineNode implementation.
-
[44 pts.] AmidaBoard implementation. Points
allotted as found in the hw5-guidelines.
- [32 pts.] There is only a
single JUnit test class that I am looking for. Note that it is actually
quite comprehensive. When testing, you should follow the basic principle
that you must first test smaller things before you test larger things. This
means that you should identify those methods (and constructors) that can be
immediately be tested.
All classes must be fully documented, according to the
JavaDoc standard introduced in class and used throughout all class examples.
Deliverables
Your goal is to turnin the
Project files by Tuesday December 5th at 10:00 AM Further details will be
posted HERE showing the preferred means of uploading your solution to the TAs.
Please be aware that no late homeworks will be accepted. This means that we will
grade as zero any homework not submitted by the above turnin means.
Notes
- [11/29/06 10:34 PM] Added Algorithm for int exitLine(int line)
- [11/28/06 9:18 AM] Homework 5 posted
©2006 George T. Heineman