IMGD 400X (B 09)
Homework Assignment #2
Bar Fly
Due by Web Turn-In: Midnight, Sunday, November 1
(See general homework instructions for turn-in details.)
The purpose of this assignment is to familiarize you with the code in Chapter 2 for state machines with messaging. You will modify the code in the WestWorld/WestWorldWithMessaging folder to add a new character into this simulation, creating his state machine and altering the Miner code to respond to him.
The new character is Sal, the Bar Fly. Sal never leaves the Saloon. His finite state machine looks like this:
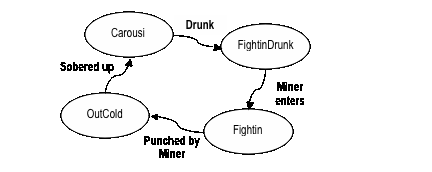
- Carousing: In the Carousing state,
Sal will raise his intoxication level by one at each update. When it reaches the drunkeness threshold of 10, he will become FightinDrunk.
- FightinDrunk: Sal stops drinking and if the Miner is in the Saloon, he will insult the Miner and begin Fightin; otherwise he waits until the Miner arrives to insult him and begin Fightin. (Sal's intoxication level does not change in this state.)
- Fightin: Sal is not much of a fighter. He never hits the Miner and one punch from the Miner knocks him out cold.
- OutCold: Sal regains sobriety while knocked out, one level per update. When he is sober (zero intoxication), he wakes up and is ready to drink some more.
Here's some implementation tips:
- Add new files to the Header Files folder in the project, BarFly.h and BarFlyOwnedStates.h, modeled on Miner.h and MinerOwnedStates.h, respectively.
- Implement BarFly.cpp in the Source Files folder, modeled on Miner.cpp.
- Implement BarFlyOwnedStates.cpp in the Source Files folder, modeled on MinerOwnedStates.cpp. Make sure to generate output on state transitions so that you can see what the agent is doing.
- Modify main.cpp to create the BarFly instance, register him as an entity, and add him to the update loop.
- Modify the Miner to do his part:
- Add a new MinerFightin state to his state machine. This can be implemented either as a state blip or as a regular transition. The entry condition should be an insult received from the BarFly and the action will be to punch the BarFly, followed by an immediate exit back to the QuenchThirst state.
- The Miner will need to send a message upon his arrival at the Saloon.
- You will need to add three messages to the MessageTypes.h:
- message when the Miner enters the saloon (this enables the BarFly to transition to the Fightin state)
- message when the BarFly insults the Miner (so the Miner can transition into his MinerFightin state)
- message one when the Miner punches (so that the BarFly can become OutCold).
What to Turn In
- Output file.
- include 'output.txt' file at toplevel of submitted zip file containing output of successful run, which includes a fight between Sal and Miner Bob.
- see cut & paste instructions in Hello West World assignment
- Modified WestWorld/WestWorldWithMessaging folder only (do not include Debug subfolder).
Grading
- 4 points - Record of successful run.
- 1 point - Software engineering quality (modularity, comments, coding style).
Please post any questions to the myWPI forum for the course.
Acknowledgement: I would like to thank Robin Burke for allowing the reuse of this assignment.